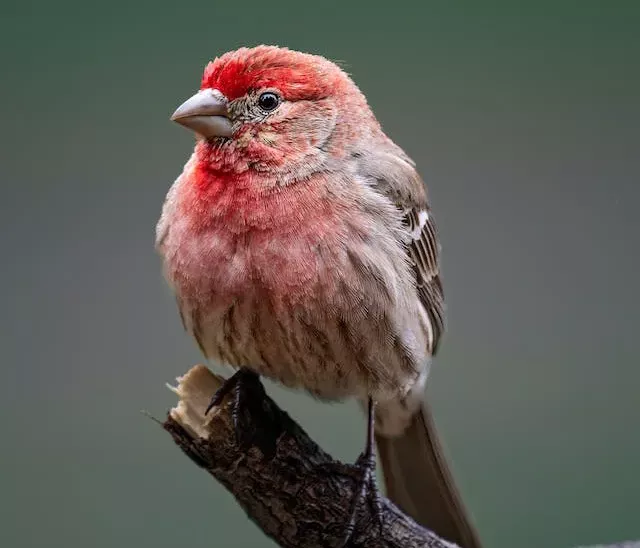
Getting a Spring Boot app to use HTTPS when running on localhost
spring https mkcert dev environment
The general process is well-outlined in this article, which helped me get started. I’ll use this post to get more into specifics of what I did and the quirks of doing this with Spring.
Creating the certificate with mkcert
At first I tried just using openssl rather than downloading mkcert, but that proved way more difficult. mkcert was as easy as it looks.
Download the pre-compiled binary
- I renamed it to just “mkcert” and put it on my Windows path so cmd could find it (I have a bin/ folder in my home folder for this kind of thing).
- Run
mkcert -install
to generate the local CA, as described in the article Create a folder to work out of (if desired) and run
mkcert local.alexandercalvert.me
as described in the web.dev article, using your own hostname instead of local.alexandercalvert.me. This creates the cert and the key as PEM files.C:\Users\alexander\local> mkcert local.alexandercalvert.me
Output:
Created a new certificate valid for the following names 📜 - "local.alexandercalvert.me" The certificate is at "./local.alexandercalvert.me.pem" and the key at "./local.alexandercalvert.me-key.pem" ✅ It will expire on 14 December 2024 🗓
Configuring the server
This StackOverflow user did the hard work of figuring this out already.
Apparently Spring Boot doesn’t have an easy way to use PEM files directly as of time of writing; it’s easiest to create a PKCS12 cert and put that into a JKS keystore.
My process was similar to the one in the StackOverflow link.
Create the PKCS12 cert from the PEM cert:
C:\Users\alexander\local> openssl pkcs12 -export -out cert.p12 -name "local-alexandercalvert-me" -inkey local.alexandercalvert.me-key.pem -in local.alexandercalvert.me.pem
- This prompted for a password. I created one with password generator.
Create a keystore containing the cert:
C:\Users\alexander\local> keytool -importkeystore -srckeystore cert.p12 -srcstoretype PKCS12 -destkeystore local-tls.jks -deststoretype JKS
This will want a password for the keystore: USE THE SAME PASSWORD YOU USED WHEN CREATING THE CERT. There’s apparently an ancient bug (or maybe unexpected assumption) in Tomcat that requires this.
To tell Spring Boot about your brand new cert, set these properties:
server.ssl.key-store-type=JKS server.ssl.key-store=C:/Users/alexander/local/local-tls.jks server.ssl.key-store-password=SECRET server.ssl.key-alias=local-alexandercalvert-me server.ssl.enabled=true server.port=8443
Tweak the appropriate values for your own scenario, of course.
In theory, that’s it - you should be able to start up your app and access it via https now on port 8443, like: https://local.alexandercalvert.me:8443.
Caveats
I found that I had to manually type https:// at the start of the url to begin with. I also got the browser warning about not trusting the cert, asking me to return to safety, etc. That shouldn’t happen if mkcert is installed, so I reran mkcert -install
and it solved both problems.
It seems PEMs might be supported soon, so the “Configuring the server” above section may become unnecessary: