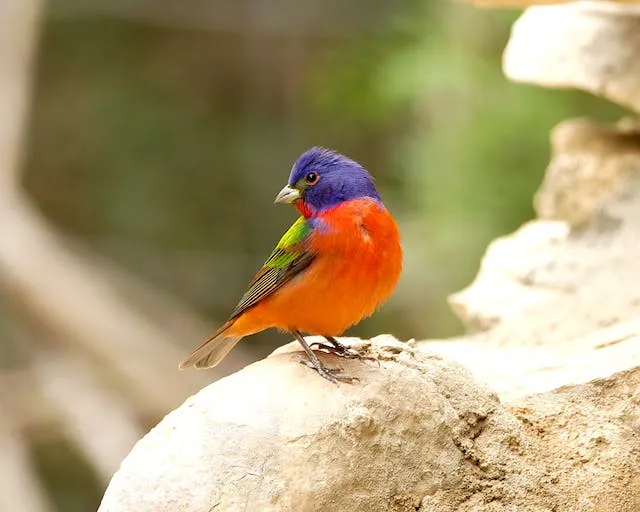
by Janice Carriger at Pexels
Colored logs with Logback
logback logging java
This will color-code your log levels in the console output, which helps to quickly identify errors and warnings as you scroll.
The overall approach is:
- Add a class that extends Logback’s
ForegroundCompositeConverterBase<ILoggingEvent>
and handles color determination logic - Add a
<conversionRule>
in your Logback XML configuration with aconversionWord
to identify it - Incorporate the conversion rule into your log pattern via the
conversionWord
Here’s my Converter implementation:
package me.alexandercalvert.logback;
import ch.qos.logback.classic.Level;
import ch.qos.logback.classic.spi.ILoggingEvent;
import static ch.qos.logback.core.pattern.color.ANSIConstants.*;
import ch.qos.logback.core.pattern.color.ForegroundCompositeConverterBase;
public class HighlightingConverter extends ForegroundCompositeConverterBase<ILoggingEvent> {
@Override
protected String getForegroundColorCode(ILoggingEvent event) {
Level level = event.getLevel();
switch (level.toInt()) {
case Level.ERROR_INT:
return BOLD + RED_FG;
case Level.WARN_INT:
return YELLOW_FG;
case Level.INFO_INT:
return GREEN_FG;
default:
return DEFAULT_FG;
}
}
}
and my logback.xml. Notice that the converterClass
attribute on the <conversionRule>
tag is the fully qualified name of the Converter implementation:
<?xml version="1.0" encoding="UTF-8"?>
<configuration scan="true">
<conversionRule conversionWord="customhighlight" converterClass="me.alexandercalvert.logback.HighlightingConverter"/>
<appender name="STDOUT" class="ch.qos.logback.core.ConsoleAppender">
<encoder>
<pattern>%d{ISO8601} %cyan([%thread]) %customhighlight(%-5level) %cyan(%logger{36}) - %msg%n</pattern>
</encoder>
</appender>
<root level="info">
<appender-ref ref="STDOUT"/>
</root>
</configuration>